💡수업 목표💡
- Javascript 문법에 익숙해진다.
- jQuery로 간단한 HTML을 조작할 수 있다.
- Ajax로 서버 API(약속)에 데이터를 주고, 결과를 받아온다.
jQuery란?
: 오픈 소스 기반의 자바스크립트 라이브러리
: HTML의 클라이언트 사이드 조작을 단순화 하도록 설계된 크로스 플랫폼의 자바스크립트 라이브러리
간단하게 말하면, JavaScript와 다른 프로그래밍 언어가 아니라 웹에서 자주 사용하는 JS 기능들을 간편하게 사용하기 위해서 미리 작성해둔 라이브러리이다. CSS선택자를 이용하기 때문에 HTML 구조를 명료하고 읽기 쉬운 형태에서 사용할수 있다는 장점이 있다.
jQuery
What is jQuery? jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers.
jquery.com
Javascript로 길고 복잡하게 써야하는 것들을
document.getElementById("element").style.display = "none";
jQuery로 보다 간단하고 직관적으로 쓸수 있다:>
$('#element').hide();
jQuery CDN
jQuery를 사용하기 위해서는 JS기능을 제공하는 라이브러리 파일과 연동해야하는데 jQuery 용량이 그렇게 크지는 않기때문에 다운받아서 사용해도 무방하지만, 네트워크를 통해서 빠르게 콘텐츠를 받을 수 있는 CDN을 주로 이용한다.
jQuery CDN
The integrity and crossorigin attributes are used for Subresource Integrity (SRI) checking. This allows browsers to ensure that resources hosted on third-party servers have not been tampered with. Use of SRI is recommended as a best-practice, whenever libr
releases.jquery.com
jQuery에는 각 버전마자 여러 jQuery파일 형태가 있다.
- uncompressed : 원본 파일 (확장자 : .js)
- minified : 원본을 압축한 파일 (확장자 : .min.js)
- slim : 해당 버전에서 필요없다고 생각하는 라이브러리를 제거한 파일 (.slim.js)
- slim minified : slim 파일을 압축한 파일(.slim.min.js)
트래픽 용량때문에 파일 용량이 적은 minified형태의 파일을 가장 많이 사용하는 편이다.
원하는 버전을 클릭하면 아래와 같은 CDN 코드가 나온다.
해당 코드를 HTML <head> </head>사이에 삽입해주면된다.
jQuery 다뤄보기
jQuery로 HTML,CSS과 마찬가지로 사용하는 모든 기능을 강의에서 다루지 않는다.
웹에 모든 움직임을 담당하는 만큼 필요할 때에 필요한 기능을 jQuery 문서를 찾아보거나 구글링해서 쓰면된다. 사실 반복해서 쓰다보면 거의 쓰는게 정해져있기때문에 걱정할 필요는 없다.
jQuery API Documentation
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. If you're new t
api.jquery.com
https://www.w3schools.com/jquery/jquery_get_started.asp
jQuery Get Started
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
input값 가져오기
// 크롬 개발자도구 콘솔창에서 쳐보기
// id 값이 url인 곳을 가리키고, val()로 값을 가져온다.
$('#url').val();
// 입력할때는?
$('#url').val('이렇게 하면 입력이 가능하지만!');
div 보이기 / 숨기기
// 크롬 개발자도구 콘솔창에 쳐보기
// id 값이 post-box인 곳을 가리키고, hide()로 안보이게 한다.
$('#post-box').hide();
// show()로 보이게 한다.
$('#post-box').show();
태그 내 html 입력하기
let temp_html = `<button>나는 추가될 버튼이다!</button>`;
$('#cards-box').append(temp_html);
// 주의: 홑따옴표(')가 아닌 backtick(`)으로 감싸야 합니다.
// 숫자 1번 키 왼쪽의 버튼을 누르면 backtick(`)이 입력됩니다.
// backtick을 사용하면 문자 중간에 Javascript 변수를 삽입할 수 있습니다.
let title = '영화 제목이 들어갑니다';
let temp_html = `<div class="col">
<div class="card h-100">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">${title}</h5>
<p class="card-text">여기에 영화에 대한 설명이 들어갑니다.</p>
<p>⭐⭐⭐</p>
<p class="mycomment">나의 한줄 평을 씁니다</p>
</div>
</div>
</div>`;
$('#cards-box').append(temp_html);
example : index.html
http://spartacodingclub.shop/web/movie
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<link href="https://fonts.googleapis.com/css2?family=Gowun+Dodum&display=swap" rel="stylesheet">
<title>스파르타코딩클럽 | 부트스트랩 연습하기</title>
<style>
* {
font-family: 'Gowun Dodum', sans-serif;
}
.myTitle {
background-color: darkgreen;
width: 100%;
height: 250px;
color: #fff;
background-image: linear-gradient(0deg, rgba(0, 0, 0, 0.5), rgba(0, 0, 0, 0.5)), url("https://movie-phinf.pstatic.net/20210715_95/1626338192428gTnJl_JPEG/movie_image.jpg");
background-position: center;
background-size: cover;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.myTitle .mybtn {
width: 200px;
height: 50px;
background-color: transparent;
color: #fff;
border-radius: 50px;
border: solid 1px #fff;
margin-top: 10px;
}
.myTitle .mybtn:hover {
border: solid 2px #fff;
}
.wrap {
width: 95%;
max-width: 1200px;
margin: 20px auto 0px;
}
.myPost {
width: 95%;
max-width: 500px;
box-shadow: 0px 0px 3px 0px gray;
padding: 20px;
margin: 20px auto;
display: none;
}
.mybtn {
display: flex;
justify-content: center;
align-items: center;
margin: 10px;
}
.mybtn button {
margin-right: 10px;
}
</style>
<script>
//01강
let count = 0;
function hey(){
count += 1;
if(count % 2 == 0){
alert("짝수입니다.");
}else{
alert("홀수입니다.");
}
}
//03강
$("#url").val("입력을 하고 싶다.");
$("#url").val();
// $("#post-box").hide();
// $("#post-box").show();
let temp_html=`<div class="col">
<div class="card">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a longer card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
<p>⭐⭐⭐</p>
<p>여기 코멘트가 들어갑니다</p>
</div>
</div>
</div>`; // ` backtick
$("#cards-box").append(temp_html);
// 04강
function open_box(){
alert("박스열기");
$("#post-box").show();
}
function close_box(){
alert("박스닫기");
$("#post-box").hide();
}
</script>
</head>
<body>
<div class="myTitle">
<h1>내 생애 최고의 영화들</h1>
<button class="mybtn" onclick="open_box();">영화 기록하기</button>
</div>
<div class="myPost" id="post-box">
<div class="form-floating mb-3">
<input type="text" class="form-control" id="url" placeholder="name@example.com">
<label for="url">영화URL</label>
</div>
<div class="input-group mb-3">
<label class="input-group-text" for="score">별점</label>
<select class="form-select" id="score">
<option selected>선택하기</option>
<option value="1">⭐</option>
<option value="2">⭐⭐</option>
<option value="3">⭐⭐⭐</option>
<option value="4">⭐⭐⭐⭐</option>
<option value="5">⭐⭐⭐⭐⭐</option>
</select>
</div>
<div class="form-floating">
<textarea class="form-control" placeholder="Leave a comment here" id="comment"></textarea>
<label for="comment">코멘트</label>
</div>
<div class="mybtn">
<button type="button" class="btn btn-dark">기록하기</button>
<button type="button" class="btn btn-outline-dark" onclick="close_box();">닫기</button>
</div>
</div>
<div class="wrap">
<div id='cards-box' class="row row-cols-1 row-cols-md-4 g-4">
<div class="col">
<div class="card">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a longer card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
<p>⭐⭐⭐</p>
<p>여기 코멘트가 들어갑니다</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a longer card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
<p>⭐⭐⭐</p>
<p>여기 코멘트가 들어갑니다</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a longer card with supporting text below as a natural lead-in to
additional content.</p>
<p>⭐⭐⭐</p>
<p>여기 코멘트가 들어갑니다</p>
</div>
</div>
</div>
<div class="col">
<div class="card">
<img src="https://movie-phinf.pstatic.net/20210728_221/1627440327667GyoYj_JPEG/movie_image.jpg"
class="card-img-top" alt="...">
<div class="card-body">
<h5 class="card-title">Card title</h5>
<p class="card-text">This is a longer card with supporting text below as a natural lead-in to
additional content. This content is a little bit longer.</p>
<p>⭐⭐⭐</p>
<p>여기 코멘트가 들어갑니다</p>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
quiz - jQuery 연습
http://spartacodingclub.shop/ajaxquiz/00_0
<!doctype html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>jQuery 연습하고 가기!</title>
<!-- JQuery를 import 합니다 -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<style type="text/css">
div.question-box {
margin: 10px 0 20px 0;
}
</style>
<script>
// 1. input-q1의 입력값을 가져온다. $('# .... ').val() 이렇게!
// 2. 만약 입력값이 빈칸이면 if(입력값=='')
// 3. alert('입력하세요!') 띄우기
// 4. alert(입력값) 띄우기
function q1() {
let q1_v = $("#input-q1").val();
if(q1_v==''){
alert('입력하세요!')
}else{
alert(q1_v);
}
}
function q2() {
// 1. input-q2 값을 가져온다.
// 2. 만약 가져온 값에 @가 있으면 (includes 이용하기 - 구글링!)
// 3. info@gmail.com -> gmail 만 추출해서 ( .split('@') 을 이용하자!)
// 4. alert(도메인 값);으로 띄우기
// 5. 만약 이메일이 아니면 '이메일이 아닙니다.' 라는 얼럿 띄우기
let q2_v = $("#input-q2").val();
if(q2_v.split('@')[1]){
alert(q2_v.split('@')[1].split(".")[0]);
}else{
alert("이메일이 아닙니다.");
}
}
function q3() {
// 1. input-q3 값을 가져온다. let txt = ... q1, q2에서 했던 걸 참고!
// 2. 가져온 값을 이용해 names-q3에 붙일 태그를 만든다. (let temp_html = `<li>${txt}</li>`) 요렇게!
// 3. 만들어둔 temp_html을 names-q3에 붙인다.(jQuery의 $('...').append(temp_html)을 이용하면 굿!)
let q3_v = $("#input-q3").val();
let temp_html = `<li>${q3_v}</li>`
$("#names-q3").append(temp_html);
}
function q3_remove() {
// 1. names-q3의 내부 태그를 모두 비운다.(jQuery의 $('....').empty()를 이용하면 굿!)
$("#names-q3").empty();
}
</script>
</head>
<body>
<h1>jQuery + Javascript의 조합을 연습하자!</h1>
<div class="question-box">
<h2>1. 빈칸 체크 함수 만들기</h2>
<h5>1-1. 버튼을 눌렀을 때 입력한 글자로 얼럿 띄우기</h5>
<h5>[완성본]1-2. 버튼을 눌렀을 때 칸에 아무것도 없으면 "입력하세요!" 얼럿 띄우기</h5>
<input id="input-q1" type="text"/>
<button onclick="q1()">클릭</button>
</div>
<hr/>
<div class="question-box">
<h2>2. 이메일 판별 함수 만들기</h2>
<h5>2-1. 버튼을 눌렀을 때 입력받은 이메일로 얼럿 띄우기</h5>
<h5>2-2. 이메일이 아니면(@가 없으면) '이메일이 아닙니다'라는 얼럿 띄우기</h5>
<h5>[완성본]2-3. 이메일 도메인만 얼럿 띄우기</h5>
<input id="input-q2" type="text"/>
<button onclick="q2()">클릭</button>
</div>
<hr/>
<div class="question-box">
<h2>3. HTML 붙이기/지우기 연습</h2>
<h5>3-1. 이름을 입력하면 아래 나오게 하기</h5>
<h5>[완성본]3-2. 다지우기 버튼을 만들기</h5>
<input id="input-q3" type="text" placeholder="여기에 이름을 입력"/>
<button onclick="q3()">이름 붙이기</button>
<button onclick="q3_remove()">다지우기</button>
<ul id="names-q3">
<li>세종대왕</li>
<li>임꺽정</li>
</ul>
</div>
</body>
</html>
서버-클라이언트 통신
웹은 크게 클라이어트와 서버로 나뉘어져서 서로 통신을 통해서 데이터를 주고 받는다. 클라이언트가 웹서비스를 통해서 데이터를 전송하고 (Request) 서버는 클라이언트가 전송한 데이터를 받아서 처리한 후 처리한 데이터를 HTML파일로 응답한다.(Response)
json이란
:JavaScript Object Notation이라는 의미ㄹ, JavaScript에서 데이터를 저장하거나 전송할때 많이 사용하는 형식이다.
JSON은, Key:Value로 이루어져 있습니다. 자료형 Dictionary와 아주- 유사하다.
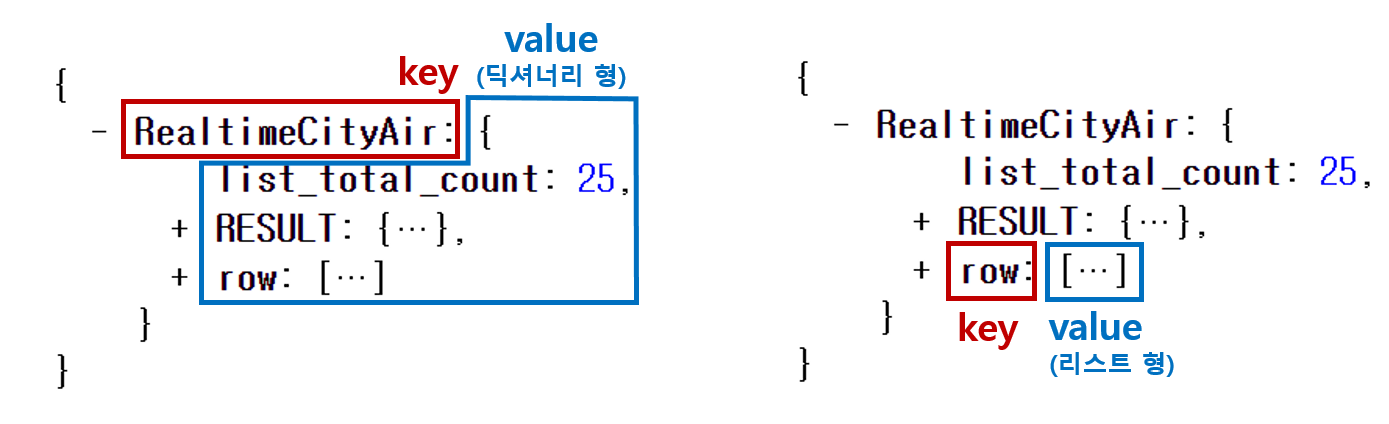
전송방법 GET/POST
API, 서버-클라이언트 통신은 은행창구와 같이 같은 예금 창구에서라도 개인이냐 기업이냐에 따라서 처리하는 방법이 다른 것처럼,클라이언트 -> 서버 로 요청할때에도 "타입"이라는 것이 존재한다.
데이터 전송방법은 HTTP 요청 메소드를 따른다. GET, POST외에도 여러가지가 있지만 네크워크적인 부분이라서 굳이 알필요 없다.
GET (정보를 가져오는 것)
: 서버에서 어떤 데이터를 가져와서 보여준다는 의미. 통상적으로 데이터 조회(Read)를 요청할때
- URL 뒤에 데이터 노출
- URL 뒤에 노출되기 때문에 글자수 제한
- URL 뒤에 노출되기 때문에 보안취약
POST(정보를 전송하는것)
: 서버의 값이나 상태를 바꾸기 위해서 사용한다는 의미.통상적으로 데이터 생성(Create), 변경(Update), 삭제(Delete) 요청 할때 사용
- URL 노출 없이 숨겨서 전송
- URL 노출이 아니라서 글자 수 제한이 없음
- URL 노출이 아니라서 보안
이번에 2주차에서는 GET을 배우고 4주차에서 POST를 배울 예정이다.
https://movie.naver.com/movie/bi/mi/basic.nhn?code=161967
위 주소는 크게 두 부분으로 쪼개진다. 바로 "?"가 쪼개지는 지점인데, "?" 기준으로 앞부분이 <서버 주소>, 뒷부분이 [영화 번호] 이다.
* 서버 주소: https://movie.naver.com/movie/bi/mi/basic.nhn
* 영화 정보: code=161967
GET방식으로 데이터를 전달할때에는
- ? : 여기서부터 전달할 데이터가 작성된다는 의미
- & : 전달할 데이터가 더 있다는 뜻
google.com/search?q=아이폰&sourceid=chrome&ie=UTF-8
위 주소는 google.com의 search 창구에 다음 정보를 전달합니다!
q=아이폰 (검색어)
sourceid=chrome (브라우저 정보)
ie=UTF-8 (인코딩 정보)
Ajax
https://api.jquery.com/jQuery.ajax/
jQuery.ajax() | jQuery API Documentation
Description: Perform an asynchronous HTTP (Ajax) request. The $.ajax() function underlies all Ajax requests sent by jQuery. It is often unnecessary to directly call this function, as several higher-level alternatives like $.get() and .load() are available
api.jquery.com
Ajax 기본 구조
$.ajax({
type: "GET", // GET 방식으로 요청한다.
url: "http://spartacodingclub.shop/sparta_api/seoulair",
data: {}, // 요청하면서 함께 줄 데이터 (GET 요청시엔 비워두세요)
success: function(response){ // 서버에서 준 결과를 response라는 변수에 담음
console.log(response) // 서버에서 준 결과를 이용해서 나머지 코드를 작성
}
})
setting는 Ajax 통신을 위한 옵션을 담고 있는 객체가 들어간다.
- type : 데이터를 전송하는 방법을 지정한다. get, post를 사용할 수 있다.
- url : 데이터를 전송하는 url을 지정한다.
- data : 서버로 데이터를 전송할 때 이 옵션을 사용한다.
- success : 성공했을 때 호출할 콜백 함수을 지정한다. Function( PlainObject data, String textStatus, jqXHR jqXHR )
성공하면, response 값에 서버의 결과값을 담아서 함수를 실행한다. - error : 실패했을 때 호출할 콜백 함수을 지정한다.
예제01 - 미세먼지 OpenAPI
http://spartacodingclub.shop/ajaxquiz/01
<!doctype html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>미세먼지 OpenAPI</title>
<!-- jQuery를 import 합니다 -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<style type="text/css">
div.question-box {
margin: 10px 0 20px 0;
}
.bad{
color:red;
}
</style>
<script>
function q1() {
// 여기에 코드를 입력하세요
$.ajax({
type: "GET",
url: "http://spartacodingclub.shop/sparta_api/seoulair",
data: {},
success: function (response) {
console.log(response)
let rows = response['RealtimeCityAir']['row'];
$("#names-q1").empty();
for (let i = 0; i < rows.length; i++) {
let gu_name = rows[i]['MSRSTE_NM'];
let gu_mise = rows[i]['IDEX_MVL'];
console.log(gu_name, gu_mise);
let temp_html;
if(gu_mise > 120){
temp_html =`<li class="bad">${gu_name} : ${gu_mise}</li>`;
}else{
temp_html =`<li>${gu_name} : ${gu_mise}</li>`;
}
$("#names-q1").append(temp_html);
}
}
})
}
</script>
</head>
<body>
<h1>jQuery+Ajax의 조합을 연습하자!</h1>
<hr/>
<div class="question-box">
<h2>1. 서울시 OpenAPI(실시간 미세먼지 상태)를 이용하기</h2>
<p>모든 구의 미세먼지를 표기해주세요</p>
<p>업데이트 버튼을 누를 때마다 지웠다 새로 씌여져야 합니다.</p>
<button onclick="q1()">업데이트</button>
<ul id="names-q1">
<!-- <li>중구 : 82</li>-->
<!-- <li>종로구 : 87</li>-->
<!-- <li>용산구 : 84</li>-->
<!-- <li>은평구 : 82</li>-->
</ul>
</div>
</body>
</html>
예제02 - 실시간 따릉이 OpenAPI
http://spartacodingclub.shop/ajaxquiz/02_2
<!doctype html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>실시간 따릉이 OpenAPI</title>
<!-- JQuery를 import 합니다 -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<style type="text/css">
div.question-box {
margin: 10px 0 20px 0;
}
table {
border: 1px solid;
border-collapse: collapse;
}
td,
th {
padding: 10px;
border: 1px solid;
}
.urgent{
color: red;
}
</style>
<script>
function q1() {
// 여기에 코드를 입력하세요
$.ajax({
type: "GET",
url: "http://spartacodingclub.shop/sparta_api/seoulbike",
data: {},
success: function (response) {
console.log(response)
let rows = response['getStationList']['row'];
$("#names-q1").empty();
for (let i = 0; i < rows.length; i++) {
let name = rows[i]['stationName'];
let rack = rows[i]['rackTotCnt'];
let bike = rows[i]['parkingBikeTotCnt'];
let temp_html;
if(bike<5){
temp_html = `<tr>
<td>${name}</td>
<td>${rack}</td>
<td class="urgent">${bike}</td>
</tr>`;
}else{
temp_html = `<tr>
<td>${name}</td>
<td>${rack}</td>
<td>${bike}</td>
</tr>`;
}
$("#names-q1").append(temp_html);
}
}
})
}
</script>
</head>
<body>
<h1>jQuery + Ajax의 조합을 연습하자!</h1>
<hr/>
<div class="question-box">
<h2>2. 서울시 OpenAPI(실시간 따릉기 현황)를 이용하기</h2>
<p>모든 위치의 따릉이 현황을 보여주세요</p>
<p>업데이트 버튼을 누를 때마다 지웠다 새로 씌여져야 합니다.</p>
<button onclick="q1()">업데이트</button>
<table>
<thead>
<tr>
<td>거치대 위치</td>
<td>거치대 수</td>
<td>현재 거치된 따릉이 수</td>
</tr>
</thead>
<tbody id="names-q1">
<tr>
<td>102. 망원역 1번출구 앞</td>
<td>22</td>
<td>0</td>
</tr>
<tr>
<td>103. 망원역 2번출구 앞</td>
<td>16</td>
<td>0</td>
</tr>
<tr>
<td>104. 합정역 1번출구 앞</td>
<td>16</td>
<td>0</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
예제03 - 랜덤 르탄이 API
http://spartacodingclub.shop/ajaxquiz/08
<!doctype html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JQuery 연습하고 가기!</title>
<!-- JQuery를 import 합니다 -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<style type="text/css">
div.question-box {
margin: 10px 0 20px 0;
}
div.question-box > div {
margin-top: 30px;
}
</style>
<script>
function q1() {
// 여기에 코드를 입력하세요
$.ajax({
type: "GET",
url: "http://spartacodingclub.shop/sparta_api/rtan",
data: {},
success: function (response) {
console.log(response)
$("#img-rtan").attr("src",response["url"]);
$("#text-rtan").text(response["msg"]);
}
})
}
</script>
</head>
<body>
<h1>JQuery+Ajax의 조합을 연습하자!</h1>
<hr/>
<div class="question-box">
<h2>3. 르탄이 API를 이용하기!</h2>
<p>아래를 르탄이 사진으로 바꿔주세요</p>
<p>업데이트 버튼을 누를 때마다 지웠다 새로 씌여져야 합니다.</p>
<button onclick="q1()">르탄이 나와</button>
<div>
<img id="img-rtan" width="300" src="http://spartacodingclub.shop/static/images/rtans/SpartaIcon11.png"/>
<h1 id="text-rtan">나는 ㅇㅇㅇ하는 르탄이!</h1>
</div>
</div>
</body>
</html>
HW02. 팬명록 날씨 API
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM"
crossorigin="anonymous"></script>
<link href="https://fonts.googleapis.com/css2?family=Gowun+Dodum&display=swap" rel="stylesheet">
<title>스파르타코딩클럽 | 세븐틴 팬명록</title>
<style>
* {
font-family: 'Gowun Dodum', sans-serif;
}
.myTitle {
background-color: darkgreen;
width: 100%;
height: 250px;
color: #fff;
background-image: linear-gradient(0deg, rgba(0, 0, 0, 0.5), rgba(0, 0, 0, 0.5)), url("https://img.hankyung.com/photo/202205/03.30135835.1.jpg");
background-position: center;
background-size: cover;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.wrap {
width: 95%;
max-width: 500px;
margin: 20px auto 0px;
}
.myPost {
width: 95%;
max-width: 500px;
box-shadow: 0px 0px 3px 0px gray;
padding: 20px;
margin: 20px auto;
}
.mybtn {
margin: 10px 0;
}
</style>
<script>
$(document).ready(function() {
alert('다 로딩됐다!');
$.ajax({
type: "GET",
url: "http://spartacodingclub.shop/sparta_api/weather/seoul",
data: {},
success: function (response) {
console.log(response)
$("#curr_temp").text(response['temp']);
}
})
});
</script>
</head>
<body>
<div class="myTitle">
<h1>세븐틴 팬명록</h1>
<p>현재기온 : <span id="curr_temp">0</span>도</p>
</div>
<div class="myPost">
<div class="form-floating mb-3">
<input type="text" class="form-control" id="floatingInput" placeholder="name@example.com">
<label for="floatingInput">닉네임</label>
</div>
<div class="form-floating">
<textarea class="form-control" placeholder="Leave a comment here" id="floatingTextarea"></textarea>
<label for="floatingTextarea">응원댓글</label>
</div>
<div class="mybtn">
<button type="button" class="btn btn-dark">응원남기기</button>
</div>
</div>
<div class="wrap">
<div class="row row-cols-1 row-cols-md-1 g-4">
<div class="col">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>사랑해요</p>
<footer class="blockquote-footer">캐럿</footer>
</blockquote>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>사랑해요</p>
<footer class="blockquote-footer">캐럿</footer>
</blockquote>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>사랑해요</p>
<footer class="blockquote-footer">캐럿</footer>
</blockquote>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>사랑해요</p>
<footer class="blockquote-footer">캐럿</footer>
</blockquote>
</div>
</div>
</div>
<div class="col">
<div class="card">
<div class="card-body">
<blockquote class="blockquote mb-0">
<p>사랑해요</p>
<footer class="blockquote-footer">캐럿</footer>
</blockquote>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
'스파르타코딩클럽 > 웹개발' 카테고리의 다른 글
웹개발종합반 개발일지 | 3주차 - 2강 (0) | 2022.08.26 |
---|---|
웹개발종합반 개발일지 | 3주차 - 1강 파이썬 설치 및 Pycharm 설정 (0) | 2022.08.26 |
웹개발종합반 개발일지 | 2주차 - 숙제 (0) | 2022.08.17 |
웹개발종합반 개발일지 | 2주차 - 6,7,8,9,10,11강 (0) | 2022.08.16 |
웹개발종합반 개발일지 | 2주차 - 1,2,3,4,5강 (0) | 2022.08.16 |